It’s well known that WordPress can get pretty bloated when you start adding various plugins and themes, it’s also pretty easy to conditionally dequeue scripts and styles so they only load when they are needed. For example, you may only need the WordPress media embed scripts on your posts so why load it on every page? The only issue with dequeuing styles and scripts is the issue of finding the handles used to enqueue then in the first place.
I wrote this super simple function that lists the enqueued handles and URLs for the scripts and styles in the frontend. Using this function you can quickly see what handles are being used and then use that handle to dequeue that style or script. It isn’t the most elegant but it’s intended for quick inspection of styles and scripts and identifying the handles.
List Enqueued Scripts and Styles Handle, WordPress Function
/** * Print enqueued style/script handles * https://lakewood.media/list-enqueued-scripts-handle/ */ function lakewood_print_scripts_styles() { if( !is_admin() && is_user_logged_in() && current_user_can( 'manage_options' )) { // Print Scripts global $wp_scripts; foreach( $wp_scripts->queue as $handle ) : echo '<div style="margin: 5px 3%; border: 1px solid #eee; padding: 20px;">Script <br />'; echo "Handle: " . $handle . '<br />'; echo "URL: " . $wp_scripts->registered[$handle]->src; echo '</div>'; endforeach; // Print Styles global $wp_styles; foreach( $wp_styles->queue as $handle ) : echo '<div style="margin: 5px 3%; border: 1px solid #eee; padding: 20px; background-color: #e0e0e0;">Style <br />'; echo "Handle: " . $handle . '<br />'; echo "URL: " . $wp_styles->registered[$handle]->src; echo '</div>'; endforeach; } } add_action( 'wp_print_scripts', 'lakewood_print_scripts_styles', 101 );
You can place the above function in your theme functions.php, I have set a few conditionals to ensure that the handles and URLs aren’t shown to public visitors and only for the logged in administrators. You can change these conditionals, you will certainly need to change the admin conditional, I’d suggest something like:
is_super_admin(1)
How is the function useful?
You can now use the handles to dequeue scripts and styles that aren’t needed on that specific page.
For example, you can use a really simple function such as below to dequeue the media embed scripts everywhere apart from single posts. You can also include the Jetpack ‘devicepx’ script from all pages and posts.
This function is also useful with plugins such as Autoptimize where you may need the script handle to make advanced changes.
function lakewood_deregister_scripts() { wp_dequeue_script('devicepx'); if( !is_single() ) { wp_dequeue_script('wp-mediaelement'); } } add_action( 'wp_print_scripts', 'lakewood_deregister_scripts', 10 );
I hope you find this article useful if you have any questions please don’t hesitate to ask in the comments below.
EDIT: Added !is_admin() to the conditional statement to prevent handles being displayed in admin.
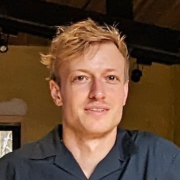
Adam
Editor of the Lakewood Journal and the founder of Lakewood media. Also an avid landscape and travel photographer.
You may also like
How to fix Webmaster Console crawl errors in WordPress
How to easily fix Google Webmaster Console Errors on your WordPress site using a simple, free plugin. Improve your WordPress site SEO.
0 Comments3 Minutes
How to Improve Your Online Presence
3 steps to improve your digital presence. Whether your business is based online or not, all companies need to have some online presence in the digital age.
0 Comments4 Minutes
How to Hunt for Affordable WordPress Consultancy Services and Actually Hire One
Learn how to find WordPress consultancy services on Google, LinkedIn, and startup directories like Beta List and Product Hunt.
0 Comments14 Minutes
How to create Legal Pages for your WordPress website
Learn how to create Legal Pages in WordPress, use manual approach, WordPress plugins, or hire a Lawyer to create Legal Pages for your WordPress site.
0 Comments13 Minutes
How to Design a Site Experience Users Will Love
It's no secret that a website is basically the face of the company. It is the representation of its story and mission, as well as the company's main point of sale, in most cases.
0 Comments12 Minutes
How to optimise and compress your images
A quick guide to optimising, compressing and resizing your images on your Mac or Windows computer before uploading to your website.
0 Comments8 Minutes